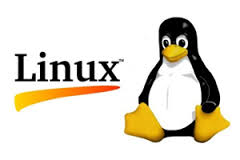
Linux Programming
List of programs
1. a) Study of Unix/Linux general purpose utility command list
man, who, cat, cd, cp, ps, ls, mv, rm, mkdir, rmdir, echo, more, date, time, kill, history, chmod, chown, finger, pwd, cal, logout, shutdown.
b) Study of vi editor.
c) Study of Bash shell, Bourne shell and C shell in Unix/Linux operating system.
d) Study of Unix/Linux file system (tree structure).
e) Study of .bashrc, /etc/bashrc and Environment variables.
2. Write a C program that makes a copy of a file using standard I/O, and system calls
3. Write a C program to emulate the UNIX ls –l command.
4. Write a C program that illustrates how to execute two commands concurrently with a command pipe.
Ex: - ls –l | sort
5. Write a C program that illustrates two processes communicating using shared memory
6. Write a C program to simulate producer and consumer problem using semaphores
7. Write C program to create a thread using pthreads library and let it run its function.
8. Write a C program to illustrate concurrent execution of threads using pthreads library.
----------------------------------------------------
1. a) Study of Unix/Linux general purpose utility command list
man, who, cat, cd, cp, ps, ls, mv, rm, mkdir, rmdir, echo, more, date, time, kill, history, chmod, chown, finger, pwd, cal, logout, shutdown.
man
Short for "manual," man allows a user to format and display the user manual built into Linux distributions, which documents commands and other aspects of the system.
Syntax
man [option(s)] keyword(s)
Example
man ls
who: identifies the users currently logged in The "who" command lets you display the users that are currently logged into your UNIX computer system. The following information is displayed: login name, workstation name, date and time of login. Entering who am i or who am I displays your login name, workstation name, date and time you logged in.
Synopsys
who [OPTION]... [ FILE | ARG1 ARG2 ]
Example
who am i
cat: concatenate or display files
Synopsys
cat [- q] [- s] [- S] [- u] [- n[- b]] [- v [- [- t] ] [- | File ... ]
The cat command reads each File parameter in sequence and writes it to standard output. If you do not specify a file name, the cat command reads from standard input. You can also specify a file name of – (minus) for standard input.
Exit Status
This command returns the following exit values:
0 All input files were output successfully.
>0 An error occurred.
Examples
1. To display a file at the workstation, enter:
cat notes
2. To concatenate several files, enter:
cat section1.1 section1.2 section1.3 >section1
3. To suppress error messages about files that do not exist, enter:
cat -q section2.1 section2.2 section2.3 >section2
4. To append one file to the end of another, enter:
cat section1.4 >>section1
The >> appends a copy of section1.4 to the end of section1. If you want to replace the file, use the >.
5. To add text to the end of a file, enter:cat >>notes
Get milk on the way home
Ctrl-D
6. To concatenate several files with text entered from the keyboard,
cat section3.1 - section3.3 >section3
7. To concatenate several files with output from another command,
li | cat section4.1 - >section4
cd:
The cd command, which stands for "change directory", changes the shell's current working directory.
Syntax
cd directory
Example
cd new
cd .
cd ..
cd
b) Study of vi editor.
How to Use the vi Editor
The vi editor is available on almost all Unix systems. vi can be used from any type of terminal
because it does not depend on arrow keys and function keys--it uses the standard alphabetic keys for commands.
vi (pronounced "vee-eye") is short for "vi"sual editor. It displays a window into the file being edited that shows 24 lines of text. vi is a text editor, not a "what you see is what you get" word processor. vi lets you add, change, and delete text, but does not provide such formatting capabilities as centering lines or indenting paragraphs.
This help note explains the basics of vi:
--> opening and closing a file
-->moving around in a file
--> elementary editing
===== Starting vi =====
You may use vi to open an already existing file by typing vi filename
where "filename" is the name of the existing file. If the file is not in your current directory, you must use the full path name.
(Or) you may create a new file by typing vi newname where "newname" is the name you wish to give the new file.
To open a new file called "testvi," enter vi testvi
On-screen, you will see blank lines, each with a tilde (~) at the left, and a line at the bottom giving the name and status of the new file:
~
~
"testvi" [New file]
===== vi Modes =====
vi has two modes:
command mode
insert mode
In command mode, the letters of the keyboard perform editing functions (like moving the cursor,
deleting text, etc.). To enter command mode, press the escape &<Esc> key.
In insert mode, the letters you type form words and sentences. Unlike many word processors, vi starts up in command mode.
===== Entering Text =====
In order to begin entering text in this empty file, you must change from command mode to insert mode. To do this, type ‘i'
Nothing appears to change, but you are now in insert mode and can begin typing text. In general, vi's commands do not display on the screen and do not require the Return key to be pressed.
Type a few short lines and press &<Return> at the end of each line. If you type a long line, you will notice the vi does not word wrap, it merely breaks the line unceremoniously at the edge of
the screen. If you make a mistake, pressing <Backspace> or <Delete> may remove the error, depending on your terminal type.
===== Moving the Cursor =====
To move the cursor to another position, you must be in command mode. If you have just finished typing text, you are still in insert mode. Go back to command mode by pressing <Esc>. If you are not sure which mode you are in, press <Esc> once or twice until you hear a beep. When you hear the beep, you are in command mode. The cursor is controlled with four keys: h, j, k, l.
Key Cursor Movement
h left one space
j down one line
k up one line
l right one space
When you have gone as far as possible in one direction, the cursor stops moving and you hear a beep. For example, you cannot use l to move right and wrap around to the next line, you must use j to move down a line. See the section entitled "Moving Around in a File" for ways to move more quickly through a file.
Basic Editing
Editing commands require that you be command mode. Many of the editing commands have a different function depending on whether they are typed as upper- or lowercase. Often, editing commands can be preceded by a number to indicate a repetition of the command.
Deleting Characters
To delete a character from a file, move the cursor until it is on the incorrect letter, then type ‘x’ The character under the cursor disappears. To remove four characters (the one under the cursor and the next three) type 4x
To delete the character before the cursor, type X (uppercase)
Deleting Words
To delete a word, move the cursor to the first letter of the word, and type dw
This command deletes the word and the space following it. To delete three words type 3dw
Deleting Lines
To delete a whole line, type dd
The cursor does not have to be at the beginning of the line. Typing dd deletes the entire line containing the cursor and places the cursor at the start of the next line. To delete two lines, type 2dd. To delete from the cursor position to the end of the line, type D (uppercase)
Replacing Characters
To replace one character with another:
1. Move the cursor to the character to be replaced.
2. Type r
3. Type the replacement character.
The new character will appear, and you will still be in command mode.
Replacing Words
To replace one word with another, move to the start of the incorrect word and type cw
The last letter of the word to be replaced will turn into a $. You are now in insert mode and may type the replacement. The new text does not need to be the same length as the original.
Press <Esc> to get back to command mode. To replace three words, type 3cw
Replacing Lines
To change text from the cursor position to the end of the line:
1. Type C (uppercase).
2. Type the replacement text.
3. Press <Esc>.
Inserting Text
To insert text in a line:
1. Position the cursor where the new text should go.
2. Type i
3. Enter the new text. The text is inserted BEFORE the cursor.
4. Press <Esc> to get back to command mode.
Appending Text
To add text to the end of a line:
1. Position the cursor on the last letter of the line.
2. Type a
3. Enter the new text. This adds text AFTER the cursor.
4. Press <Esc> to get back to command mode.
Opening a Blank Line
To insert a blank line below the current line, type o (lowercase)
To insert a blank line above the current line, type O (uppercase)
Joining Lines
To join two lines together:
1. Put the cursor on the first line to be joined.
2. Type J
To join three lines together:
1. Put the cursor on the first line to be joined.
2. Type 3J
===== Undoing =====
To undo your most recent edit, type u
To undo all the edits on a single line, type U (uppercase)
Undoing all edits on a single line only works as long as the cursor stays on that line. Once you move the cursor off a line, you cannot use U to restore the line.
=== Moving Around in a File ==
There are shortcuts to move more quickly though a file. All these work in command mode.
Key Movement
w forward word by word
b backward word by word
$ to end of line
0 (zero) to beginning of line
H to top line of screen
M to middle line of screen
L to last line of screen
G to last line of file
1G to first line of file
<Control>f scroll forward one screen
<Control>b scroll backward one screen
<Control>d scroll down one-half screen
<Control>u scroll up one-half screen
== Moving by Searching ==
To move quickly by searching for text, while in command mode:
1. Type / (slash).
2. Enter the text to search for.
3. Press <Return>.
The cursor moves to the first occurrence of that text.
To repeat the search in a forward direction, type n
To repeat the search in a backward direction, type N
== Closing and Saving a File ==
With vi, you edit a copy of the file, rather than the original file. Changes are made to the original only when you save your edits.
To save the file and quit vi, type ZZ
The vi editor is built on an earlier Unix text editor called ex. ex commands can be used with in vi. ex commands begin with a : (colon) and end with a <Return>. The command is displayed on the status line as you type. Some ex commands are useful when saving and closing files.
To save the edits you have made, but leave vi running and your file open:
1. Press <Esc>.
2. Type :w
3. Press <Return>.
To quit vi, and discard any changes your have made since last saving:
1. Press <Esc>.
2. Type :q!
3. Press <Return>.
c) Study of Bash shell, Bourne shell and C shell in Unix/Linux operating system.
Types of Shells in Linux
In addition to graphical user interfaces like Gnome, KDE and MATE, the Linux operating system also offers several shells. These command-line interfaces provide powerful environments
for software development and system maintenance. Though shells have many commands in common, each type has unique features. Over time, individual programmers come to prefer one type of shell over another; some develop new, enhanced shells based on previous ones. UNIX also has an ecosystem of different shells; Linux carries this practice into the open-source software arena.
The Bourne shell
The Bourne shell, called "sh," is one of the original shells, developed for Unix computers by Stephen Bourne at AT&T's Bell Labs in 1977. Its long history of use means many software developers are familiar with it. It offers features such as input and output redirection, shell scripting with string and integer variables, and condition testing and looping.
The Bash shell
The popularity of sh motivated programmers to develop a shell that was compatible with it, but with several enhancements. Linux systems still offer the sh shell, but "bash" -- the "Bourne-again Shell," based on sh -- has become the new default standard. One attractive feature of bash is its ability to run sh shell scripts unchanged. Shell scripts are complex sets of commands that automate programming and maintenance chores; being able to reuse these scripts saves programmers time. Conveniences not present with the original Bourne shell include command completion and a command history.
C Shell
Developers have written large parts of the Linux operating system in the C and C++ languages.
Using C syntax as a model, Bill Joy at Berkeley University developed the "C-shell," csh, in 1978. Ken Greer, working at Carnegie-Mellon University, took csh concepts a step forward with a new shell, tcsh, which Linux systems now offer. Tcsh fixed problems in csh and addedcommand completion, in which the shell makes educated "guesses" as you type, based on your
system's directory structure and files. Tcsh does not run bash scripts, as the two have substantial
differences.
The Korn shell
David Korn developed the Korn shell, or ksh, about the time tcsh was introduced. Ksh is
compatible with sh and bash. Ksh improves on the Bourne shell by adding floating-point
arithmetic, job control, and command aliasing and command completion. AT&T held proprietary
rights to ksh until 2000, when it became open source.
d) Study of Unix/Linux file system (tree structure).
A file system is a logical collection of files on a partition or disk
UNIX uses a hierarchical file system structure, much like an upside-down tree, with root (/) at
the base of the file system and all other directories spreading from there.
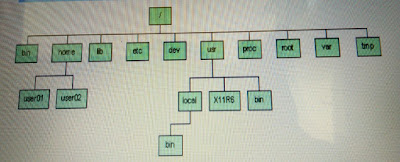
A UNIX filesystem is a collection of files and directories that has the following properties −
-->It has a root directory (/) that contains other files and directories.
--> Each file or directory is uniquely identified by its name, the directory in which it resides, and a unique identifier, typically called an inode.
-->By convention, the root directory has an inode number of 2 and the lost+found directory has an inode number of 3. Inode numbers 0 and 1 are not used. File inode numbers can be seen by specifying the -i option to ls command.
--> It is self contained. There are no dependencies between one filesystem and any other.
*The directories have specific purposes and generally hold the same types of information for easily locating files. Following are the directories that exist on the major versions of Unix −
Directory Description
/ This is the root directory which should contain only the directories needed at the top level of the file structure.
/bin This is where the executable files are located. They are available to all user.
/dev These are device drivers.
/etc Supervisor directory commands, configuration files, disk configuratio files,valid user lists, groups, ethernet, hosts, where to send critical messages.
/lib Contains shared library files and sometimes other kernel-related files.
/boot Contains files for booting the system.
/home Contains the home directory for users and other accounts.
/mnt Used to mount other temporary file systems, such as cdrom and floppy for the CD-ROM drive and floppy diskette drive, respectively
/proc Contains all processes marked as a file by process number or other information that is dynamic to the system.
/tmp Holds temporary files used between system boots
/usr Used for miscellaneous purposes, or can be used by many users. Includes
administrative commands, shared files, library files, and others
/var Typically contains variable-length files such as log and print files and any other
type of file that may contain a variable amount of data
/sbin Contains binary (executable) files, usually for system administration. For
example fdisk and ifconfig utlities.
/kernel Contains kernel files
e) Study of .bashrc, /etc/bashrc and Environment variables.
Following is the partial list of important environment variables.
Variable Description
DISPLAY - Contains the identifier for the display that X11 programs should use by default.
HOME - Indicates the home directory of the current user: the default argument for the cd built-in command.
IFS - Indicates the Internal Field Separator that is used by the parser for word splitting after expansion.
LANG - LANG expands to the default system locale; LC_ALL can be used to override this. For example, if its value is pt_BR, then the language is set to (Brazilian) Portuguese and the locale to Brazil.
LD_LIBRARY_PATH - On many Unix systems with a dynamic linker, contains a colon-
separated list of directories that the dynamic linker should search
for shared objects when building a process image after exec,before searching in any other directories.
PATH - Indicates search path for commands. It is a colon-separated list of directories in which the shell looks for commands
PWD - Indicates the current working directory as set by the cd command.
RANDOM - Generates a random integer between 0 and 32,767 each time it is referenced.
SHLVL - Increments by one each time an instance of bash is started. This variable is useful for determining whether the built-in exit command ends the current session.
TERM - Refers to the display type
TZ - Refers to Time zone. It can take values like GMT, AST, etc.
UID - Expands to the numeric user ID of the current user, initialized at shell startup.
2. Write a C program that makes a copy of a file using standard I/O, and system calls.
2.a)
Aim: Write a C program that makes a copy of a file using standard I/O
#include <stdio.h>
int main(int argc, char *argv[])
{
FILE *fp1,*fp2;
int c;
if(argc<2)
{
printf("No arguments passed\n");
return;
}
fp1 = fopen(argv[1], "r"); /* open existing file */
fp2 = fopen(argv[2], "w"); /* open new file */
while ((c=fgetc(fp1)) != EOF)
fputc(c,fp2);
fclose(fp1);
fclose(fp2);
}
2.b)
Aim: Write a C program that makes a copy of a file using system calls.
#include <stdio.h>
#include <fcntl.h>
#include<stdlib.h>
#define PERMS 0666 /* RW for owner, group, others */
#define BUFF 1024
/* cp: copy f1 to f2 */
main(int argc, char *argv[])
{
int f1, f2, n;
char buf[BUFF];
if (argc != 3)
{
perror("Error: please enter two arguments");
exit(0);
}
if ((f1 = open(argv[1], O_RDONLY, 0)) == -1)
{
perror("can't open");
exit(0);
}
if ((f2 = creat(argv[2], PERMS)) == -1)
{
perror("can't create permissions");
exit(0);
}
while ((n = read(f1, buf, BUFF)) > 0)
if (write(f2, buf, n) != n)
perror("write error on file ");
printf("File copied successfully");
return 0;
}
3. Write a C program to emulate the UNIX ls –l command.
#include <unistd.h>
#include <stdio.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <stdlib.h>
int main(int argc, char **argv)
{
if(argc != 2)
{
perror("Enter a file name");
exit(0);
}
struct stat fileStat;
if(stat(argv[1],&fileStat) < 0)
{
perror("File Error");
exit(0);
}
printf("Information for %s\n",argv[1]);
printf("---------------------------\n");
printf("File Size: \t\t%lu bytes\n",fileStat.st_size);
printf("Number of Links: \t%d\n",fileStat.st_nlink);
printf("File inode: \t\t%lu\n",fileStat.st_ino);
printf("File Permissions: \t");
printf( (S_ISDIR(fileStat.st_mode)) ? "d" : "-");
printf( (fileStat.st_mode & S_IRUSR) ? "r" : "-");
printf( (fileStat.st_mode & S_IWUSR) ? "w" : "-");
printf( (fileStat.st_mode & S_IXUSR) ? "x" : "-");
printf( (fileStat.st_mode & S_IRGRP) ? "r" : "-");
printf( (fileStat.st_mode & S_IWGRP) ? "w" : "-");
printf( (fileStat.st_mode & S_IXGRP) ? "x" : "-");
printf( (fileStat.st_mode & S_IROTH) ? "r" : "-");
printf( (fileStat.st_mode & S_IWOTH) ? "w" : "-");
printf( (fileStat.st_mode & S_IXOTH) ? "x" : "-");
printf("\n\n");
printf("The file %s a symbolic link\n", (S_ISLNK(fileStat.st_mode)) ? "is" : "is not");
return 0;
}
4. Write a C program that illustrates how to execute two commands concurrently with a command pipe.
Program:
#include<stdio.h>
#include<stdlib.h>
int main(int argc,char *argv[])
{
int fd[2],pid,k;
k=pipe(fd);
if(k==-1)
{
perror("pipe");
exit(1);
}
pid=fork();
if(pid==0)
{
close(fd[0]);
dup2(fd[1],1);
close(fd[1]);
execlp(argv[1],argv[1],NULL);
perror("execl");
}
else
{
wait(2);
close(fd[1]);
dup2(fd[0],0);
close(fd[0]);
execlp(argv[2],argv[2],NULL);
perror("execl");
}
}
Output:
$./a.out ls sort
a.out
ls_sort.c
named.
pip
prog.
read.
typescript
unnamed.c
write.c
5. Write a C program that illustrates two processes communicating using shared memory
For a server, it should be started before any client. The server should perform
the following tasks:
1. Ask for a shared memory with a memory key and memorize the returned
shared memory ID. This is performed by system call shmget().
2. Attach this shared memory to the server's address space with system
call shmat().
3. Initialize the shared memory, if necessary.
4. Do something and wait for all clients' completion.
5. Detach the shared memory with system call shmdt().
6. Remove the shared memory with system call shmctl().
For the client part, the procedure is almost the same:
1. Ask for a shared memory with the same memory key and memorize the
returned shared memory ID.
2. Attach this shared memory to the client's address space.
3. Use the memory.
4. Detach all shared memory segments, if necessary.
5. Exit.
shm_server.c
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <stdio.h>
#include <stdlib.h>
#define SHMSZ 27
main()
{
char c;
int shmid;
key_t key;
char *shm, *s;
/*
* We'll name our shared memory segment
* "5678".
*/
key = 5678;
/*
* Create the segment.
*/
if ((shmid = shmget(key, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
/*
* Now we attach the segment to our data space.
*/
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
/*
* Now put some things into the memory for the
* other process to read.
*/
s = shm;
for (c = 'a'; c <= 'z'; c++)
*s++ = c;
*s = NULL;
/*
* Finally, we wait until the other process
* changes the first character of our memory
* to '*', indicating that it has read what
* we put there.
*/
while (*shm != '*')
sleep(1);
exit(0);
}
shm_client.c
/*
* shm-client - client program to demonstrate shared memory.
*/
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <stdio.h>
#include <stdlib.h>
#define SHMSZ 27
main()
{
int shmid;
key_t key;
char *shm, *s;
/*
* We need to get the segment named
* "5678", created by the server.
*/
key = 5678;
/*
* Locate the segment.
*/
if ((shmid = shmget(key, SHMSZ, 0666)) < 0) {
perror("shmget");
exit(1);
}
/*
* Now we attach the segment to our data space.
*/
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
/*
* Now read what the server put in the memory.
*/
for (s = shm; *s != NULL; s++)
putchar(*s);
putchar('\n');
/*
* Finally, change the first character of the
* segment to '*', indicating we have read
* the segment.
*/
*shm = '*';
exit(0);
}
6. Write a C program to simulate producer and consumer problem using semaphores
ALGORITHM:
1. Start the process
2. Initialize buffer size
3. Consumer enters, before that producer buffer was not empty.
4. Producer enters, before check consumer consumes the buffer.
5. Stop the process.
PROGRAM:
#include<stdio.h>
int mutex=1,full=0,empty=3,x=0;
main()
{
int n;
void producer();
void consumer();
int wait(int);
int signal(int);
printf("\n1.PRODUCER\n2.CONSUMER\n3.EXIT\n");
while(1)
{
printf("\nENTER YOUR CHOICE\n");
scanf("%d",&n);
switch(n)
{
case 1:
if((mutex==1)&&(empty!=0))
producer();
else
printf("BUFFER IS FULL");
break;
case 2:
if((mutex==1)&&(full!=0))
consumer();
else
printf("BUFFER IS EMPTY");
break;
case 3:
exit(0);
break;
}
}
}
int wait(int s)
{
return(--s);
}
int signal(int s)
{
return(++s);
}
void producer()
{
mutex=wait(mutex);
full=signal(full);
empty=wait(empty);
x++;
printf("\nproducer produces the item%d",x);
mutex=signal(mutex);
}
void consumer()
{
mutex=wait(mutex);
full=wait(full);
empty=signal(empty);
printf("\n consumer consumes item%d",x);
x--;
mutex=signal(mutex);
}
OUTPUT:
[root@localhost ~]$ ./a.out
1.PRODUCER
2.CONSUMER
3.EXIT
ENTER YOUR CHOICE
1
producer produces the item1
ENTER YOUR CHOICE
1
producer produces the item2
ENTER YOUR CHOICE
2
consumer consumes item2
ENTER YOUR CHOICE
2
consumer consumes item1
ENTER YOUR CHOICE
2
BUFFER IS EMPTY
ENTER YOUR CHOICE
3
No comments:
Post a Comment